GraphQL vs. REST

GraphQL and REST are two common architectural patterns for building APIs. REST APIs use standard HTTP methods to access resources through dedicated endpoints, while GraphQL APIs let clients query the exact data they need through a single endpoint, which helps prevent over-fetching. According to Postman’s 2023 State of the API report, 86% of developers use REST, while 29% use GraphQL.
In this article, we will review GraphQL and REST and discuss their benefits, similarities, and differences.
What is REST?
REST, which stands for Representational State Transfer, is an architectural style that provides a simple and uniform interface for sharing resources between a client and a server. REST defines specific operations that use standard HTTP methods to perform CRUD (Create, Read, Update, Delete) actions on resources.
A REST API must satisfy the following constraints:
- Client-server architecture: The client and server should be separate, allowing each to evolve independently. The client is responsible for the user interface and user experience, while the server is responsible for storing, processing, and retrieving data. This separation improves scalability and portability.
- Stateless operations: Every client request must contain all the information the server needs to understand and process the request. The server should not have to store any context about the client session between requests, which facilitates scalability and improves reliability.
- RESTful resource caching: Responses from the server can be labeled as cacheable or non-cacheable. If a response is cacheable, the client can reuse it for similar requests in the future. Effective caching practices can reduce the number of interactions between the client and server.
- Layered system: A REST API can consist of multiple layers, each with a specific functionality. For example, an e-commerce API can have separate layers for authentication, fetching product information, and processing orders. A client interacts with a layer without necessarily knowing what other layers exist or what their functions are. This layered architecture allows for increased flexibility and easier management, as individual layers can evolve or be replaced independently.
- Use of a uniform interface (UI): The REST architecture emphasizes that all of an API’s interactions should be uniform and predictable. The uniform interface is defined by a set of conventions, which includes the use of standard HTTP methods and the identification of resources using URIs.
- Code on demand: This is the only optional constraint in REST. It allows the server to extend the functionality of a client by sending it executable code. For example, a server can send JavaScript code to a client to be executed in a web browser.
What are the benefits of working with REST APIs?
benefits that make it easier for developers to build and work with efficient and scalable APIs. These benefits include:
- Ease of use: Developers already familiar with HTTP can pick up REST easily. It uses standard HTTP methods and paradigms, which gives it a very familiar flow.
- Language agnosticism: REST APIs can be implemented in any language that supports HTTP without needing any additional support or communication layer.
- Strong community and tooling: REST is very popular and has been around for over three decades. It has a strong community backing, and several developers have invested time and resources into building robust tooling that makes it easier to work with REST APIs.
- Uniform interface: The uniformity of the REST architecture ensures a consistent and predictable interface, simplifying interactions and integrations between different systems.
What is GraphQL?
GraphQL is a query language for APIs and a server-side runtime for executing queries using a type system you define for your data. GraphQL exposes a single endpoint for querying, updating, creating, and deleting data, and it allows clients to request and receive the specific data they need—without having to chain requests together.
GraphQL uses HTTP as its transport protocol, and it enables you to perform three operation types: queries, mutations, and subscriptions.
- Queries: Queries allow the client to request the specific data it needs using a defined data schema. They can be used to fetch data from multiple data sources—or even other APIs—without the need to make multiple round-trip calls to the server.
- Mutations: Mutations in GraphQL allow the client to mutate data on the server. This includes creating or updating existing data objects.
- Subscriptions: Subscriptions facilitate bi-directional, real-time communication between the client and the server. The client can subscribe to events and get updated as soon as these events are published, or vice versa.
What are the benefits of GraphQL?
GraphQL was initially developed to solve some of the limitations of REST APIs, such as over-fetching. It also provides an elegant way to request and manipulate data. Some of the benefits of GraphQL include:
- Single endpoint: GraphQL provides a single endpoint for all operations. This simplifies the structure of the API’s URL and eliminates the need to maintain multiple paths or routes in an API.
- Flexible data retrieval: GraphQL provides a mechanism that allows clients to specify and receive the exact data they need. This reduces the risk of over-fetching and under-fetching data and improves the efficiency of network requests, since only necessary data objects are transmitted.
- Strongly typed schema: GraphQL requires a schema that defines the data types and relationships between each type. The schema provides clients with a description of the data that is available to them.
- Support for introspection: GraphQL APIs are self-documenting. Clients can query the API schema to discover the types, queries, mutations, and fields it supports. This introspective capability makes it easier to understand and explore the capabilities of a GraphQL API.
What are the similarities between GraphQL and REST?
While GraphQL and REST differ in their design philosophies, they are both used for building APIs for web services and therefore have many similarities. Some of these similarities include:
- Common architectural principles: GraphQL and REST share common architectural principles. They are both stateless, support a client-server model, and can be consumed by any client that understands HTTP. This makes them suitable for a wide range of devices and applications.
- HTTP as transport layer: GraphQL and REST typically use HTTP as their transport layer, and they both leverage the standard HTTP POST method to send and receive data.
- Data transfer formats: GraphQL and REST use standard data formats for communication. JSON is the most commonly used format, but other formats, like XML, can also be used, especially in REST.
- Middleware and extensions: GraphQL and REST can both be extended with middleware to add functionalities like logging, caching, and authentication.
What are the differences between GraphQL and REST?
GraphQL and REST have several differences in their design patterns and approach to data modeling. These differences include:
Enforced schema
- GraphQL enforces the use of a schema—regardless of whether developers are taking a schema-first or code-first approach. With the code-first approach, the schema is generated from the resolvers.
- REST does not enforce the use of a schema. Developers can take a schema-first approach with REST by using API specifications like OpenAPI and AsyncAPI, or they can auto-generate schemas from code, but it is not an integral part of the REST architecture.
Popularity
- GraphQL was released by Facebook in 2015. It has been rapidly growing since then and has gained a lot of popularity over the last few years. According to Postman’s State of the API report, 29% of developers make use of GraphQL.
- REST has been widely used for several decades and is a well-established standard for building APIs—especially public ones. According to the State of the APIs report, 90% of developers use REST APIs.
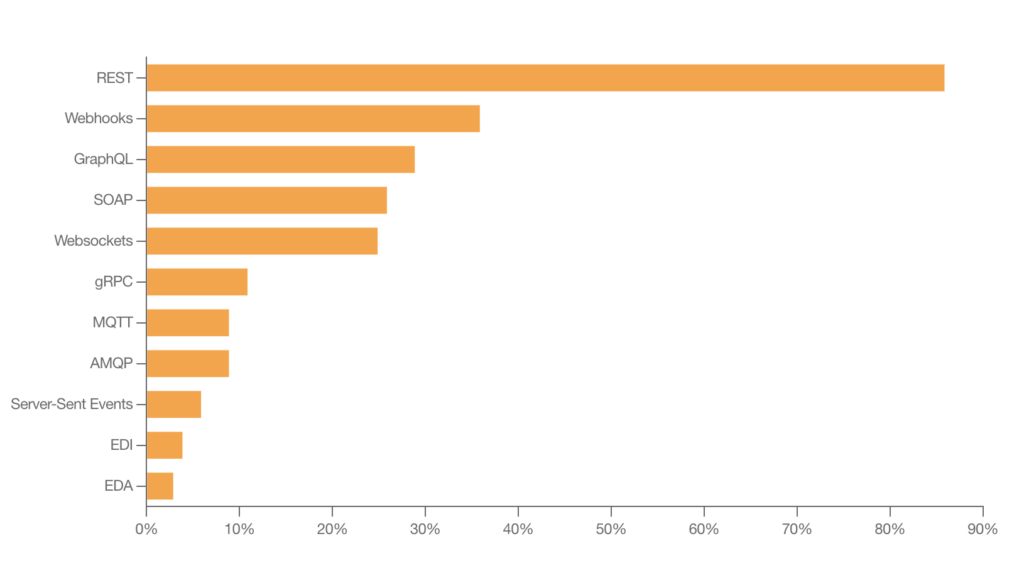
HTTP status codes
- GraphQL uses the 200 status code for all responses, including error responses. The error response is typically included in the response payload itself.
- REST makes use of standard HTTP status codes that indicate the status of the response. This is very useful for error handling.
Versioning
- GraphQL uses a single versioned endpoint. It handles changes and deprecations by updating and evolving the schema without explicitly versioning the API, which can promote a smoother long-term evolution.
- REST APIs need to be versioned in order to safely handle changes and deprecation. This is usually done in the URL of the APIs, though there are several other approaches to API versioning, as well.
Performance
- GraphQL gives clients lots of flexibility in querying data, which makes it more efficient. Clients can request and receive the exact data they want—and nothing else. This means that unnecessary data is not transmitted, and clients don’t have to send more than one request to get the data they need.
- Depending on how the API was built, REST APIs run the risk of over-fetching or under-fetching data. Clients may need to send multiple requests to get the data they need.
Introspection
- GraphQL supports introspection, which allows clients to easily retrieve relevant details about the schema. Introspection can help developers automatically generate queries and documentation—and explore the different queries, mutations, and subscriptions that can be performed.
- REST APIs typically do not have built-in support for introspection. However, API specifications like OpenAPI or AsyncAPI make introspection possible and offer the same benefits as that of a GraphQL schema.
The article tends to lean towards REST being less effective than GraphQL. I think that’s only true if the REST API is designed that way or the consumption of the api is incorrect.
The same could be said for flexibility. You can build APIs that return dynamic data based on queries without making use of GraphQL.
Also, spamming of GraphQL leads to significant overhead as your reaching into multiple backend services.
It’s important to note that GraphQL solves a very specific problem, multi dataset queries. But saying that it’s more efficient…. I guess that’s a matter of design right?